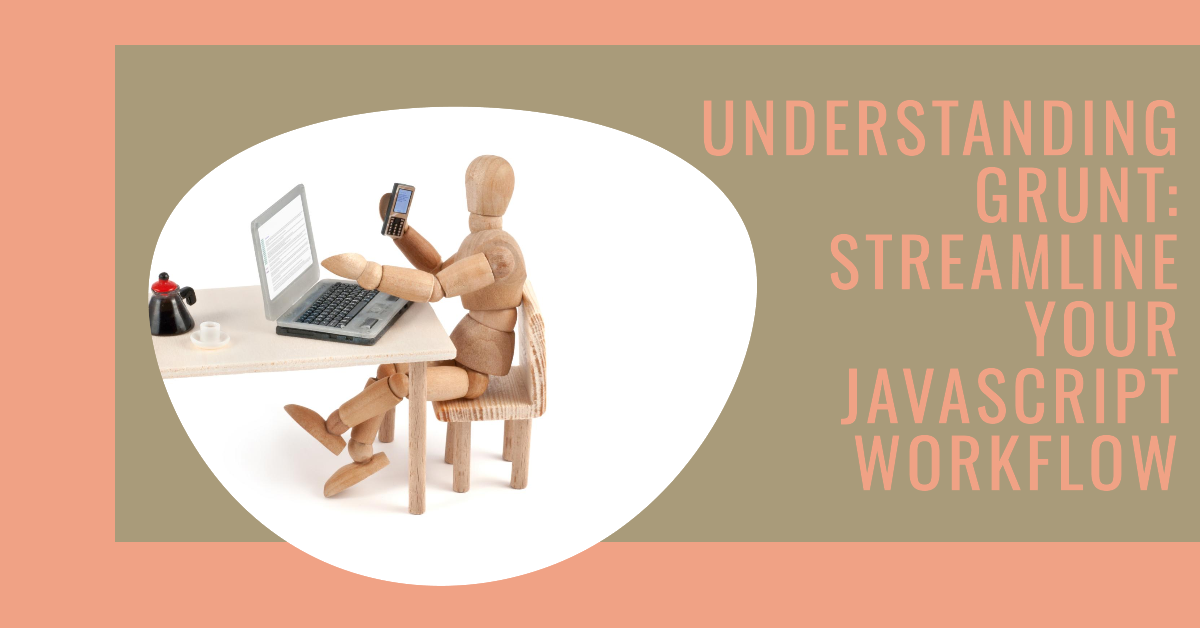
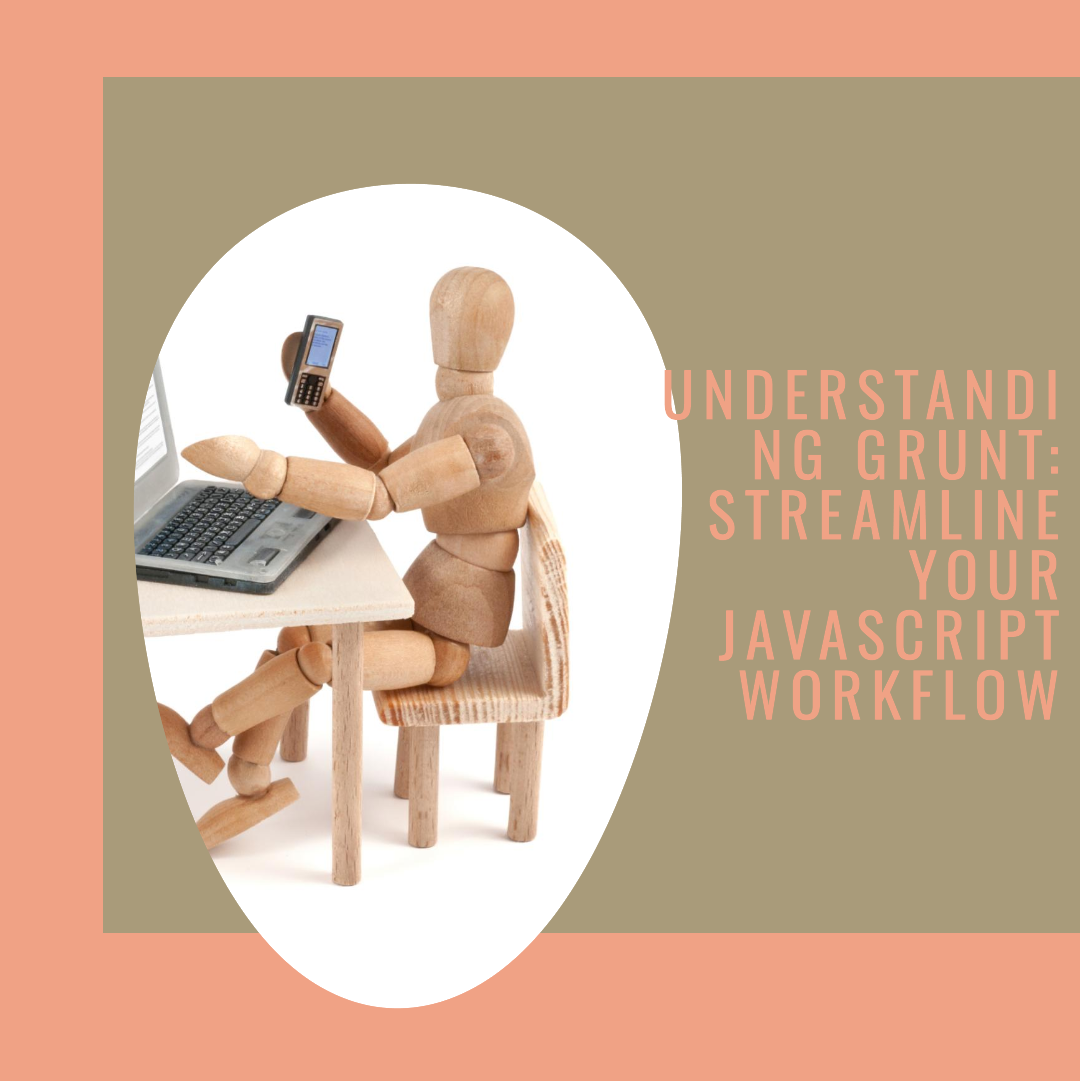
Grunt is a powerful JavaScript task runner and build automation tool that simplifies the process of performing repetitive tasks in web development. It allows developers to automate tasks such as minification, compilation, unit testing, and more, thereby enhancing productivity and reducing manual effort. This article delves into what Grunt is, its key features, practical examples of usage, benefits, and how it can optimize your JavaScript development workflow.
What is Grunt?
Grunt is a JavaScript task runner that automates repetitive tasks involved in web development. It operates via plugins that provide specific functionalities, such as compiling Sass to CSS, concatenating JavaScript files, optimizing images, and running tests. Grunt's configuration is defined in a Gruntfile.js, where tasks and plugins are specified.
Key Features of Grunt:
Benefits of Using Grunt:
Getting Started with Grunt:
Start by installing Grunt globally via npm:
npm install -g grunt-cli
Create a package.json file in your project directory (if not already present):
npm init -y
Install Grunt and necessary plugins as local dependencies:
npm install grunt --save-dev
npm install grunt-contrib-uglify grunt-contrib-sass --save-dev
Create a Gruntfile.js in the root of your project directory. Define tasks, load plugins, and configure task options:
module.exports = function(grunt) {
grunt.initConfig({
uglify: {
options: {
mangle: false
},
my_target: {
files: {
'dist/app.min.js': ['src/app.js']
}
}
},
sass: {
dist: {
files: {
'dist/style.css': 'src/style.scss'
}
}
}
});
// Load plugins
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-contrib-sass');
// Define default task(s)
grunt.registerTask('default', ['uglify', 'sass']);
};
Execute Grunt tasks using the grunt command in your terminal:
grunt
Practical Examples:
grunt.initConfig({
uglify: {
options: {
mangle: false
},
my_target: {
files: {
'dist/app.min.js': ['src/app.js']
}
}
}
});
grunt.initConfig({
sass: {
dist: {
files: {
'dist/style.css': 'src/style.scss'
}
}
}
});
grunt.initConfig({
watch: {
scripts: {
files: ['src/*.js'],
tasks: ['uglify'],
options: {
spawn: false,
},
},
styles: {
files: ['src/*.scss'],
tasks: ['sass'],
options: {
spawn: false,
},
},
},
});
In conclusion, Grunt is a valuable tool for JavaScript developers seeking to automate and streamline their development workflows. By leveraging Grunt's task automation capabilities, developers can improve productivity, ensure code quality, optimize performance, and customize build processes according to project requirements. Whether you're working on a small website or a complex web application, mastering Grunt will empower you to build better and more efficient JavaScript projects. Stay updated with Grunt's ecosystem and best practices to maximize its potential in your development toolkit.